node全域物件:global
node的全域物件為 global ,就像網頁的全域物件為 window。
如下圖,在node環境下,不同js檔之間是區隔的,使用到相同的變數名稱是不會互相影響。
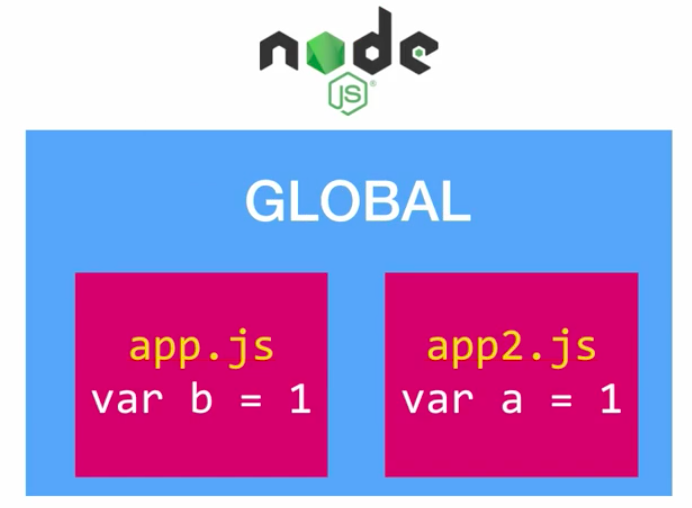
require、module exports
由於node環境下,不同js檔之間是區隔的,所以若想要在js檔之間達到資料的傳遞的話,方法如下
1 | //引用 模組 |
輸出語法有二種,可任意選擇喜歡的寫法,但不能二種寫法同時在同一個js檔裡,因為會互相覆蓋掉。
1 |
|
Node核心模組-createServer
createServer的參數:
request:接收User的行為,如:request.url 可取得目前網頁網址
response:回應User的結果
1 | var http = require('http'); |
常見的port:
21 FTP
80 http
3389 遠端桌面
21 FTP
80 http
3389 遠端桌面
Node模組-Path
__dirname:取得目前js檔的目錄路徑,如:/Users/kanboo/Desktop/project
__filename:取得目前js檔的檔案路徑,如:/Users/kanboo/Desktop/project/app.js
1 | var path = require('path'); |
NPM-nodemon
nodemon是一個專為Node.js設計的模組,它的作用是持續監視著你的程式碼,一旦你修改後保存了,nodemon就會重新啟動你的Node.js程式,這樣你只要刷新你的瀏覽器就能看到改動。
1 | npm install -g nodemon |
1 | //原先啟動server的方法 |