按下鍵盤時,觸發聲音及特效
目標
- 按下鍵盤時,播放聲音、顯示CSS效果
- 播放完畢後,移除CSS效果
實踐步驟
先監聽 鍵盤 的
keydown
Event- 利用
e.keyCode
取得符合的 audio標籤 和 div標籤 - audio 標籤 => 播放 聲音
- div 標籤 => 顯示 CSS效果
- 利用
監聽 CSS 的
transitionend
Event (transitionend 事件會在 transition 结束后觸發)e.propertyName !== "transform"
僅針對 transform 繼續做事,不是則停止- 若為 transform,則移除 CSS效果
成品
JS學習紀錄
HTML5標籤 HTMLMediaElement
透過 js 取得 HTMLMediaElement 元素,來進行影音的播放。
1 | <audio data-key="65" src="sounds/clap.wav"></audio> |
1 | //取得 HTMLMediaElement 元素 |
DOM元素 Element.classList
透過 classList
新增、移除、切換 CSS屬性,就像jQuery的 addClass
、removeClass
一樣。
1 | <div data-key="65" class="key"> |
1 | //取得 DOM 元素 |
DOM元素 NodeList
使用 querySelectorAll
取得的DOM,返回的結果是 NodeList
型態,非 Array 型態,所以不能使用 Array的method,若要使用Array的method的話,就需轉換成Array型態。
1 | <div data-key="65" class="key"> |
querySelectorAll 取得 DOM元素後,使用 NodeList.prototype.forEach() 的 method,有可能會在部份瀏覽器不支援。
1 | // 取得 DOM 元素 => NodeList 型態 |
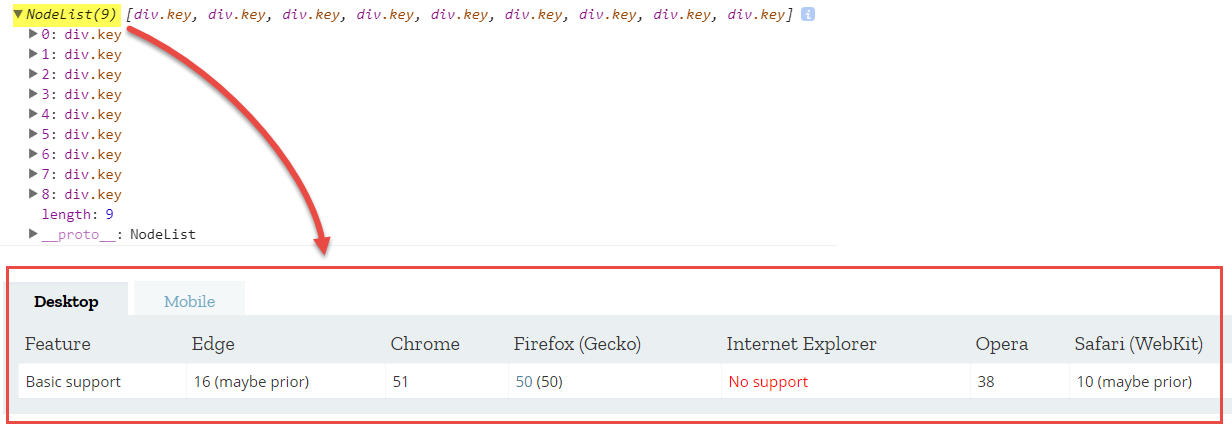
可藉由下列方法,將 NodeList 轉換 Array型態,支援度較高。
1 | // 第一種方法:Array.from() |
CSS監聽事件 transitionend
透過 CSS transitionend
Event ,當 transition 效果結束後,就會觸發此事件,
1 | function removeTransition(e){ |
圖1:同時多個 CSS效果
圖2:event.propertyName 取得 CSS屬性名稱
一般函式的this(作為 DOM 事件偵聽函式 → 該 DOM)
在 removeTransition
function裡面有使用 this
去移除CSS屬性,該如何判斷這個 this
是指向誰呢?
答案是:一般函式的this(作為 DOM 事件偵聽函式 → 該 DOM)
1 | function removeTransition(e){ |